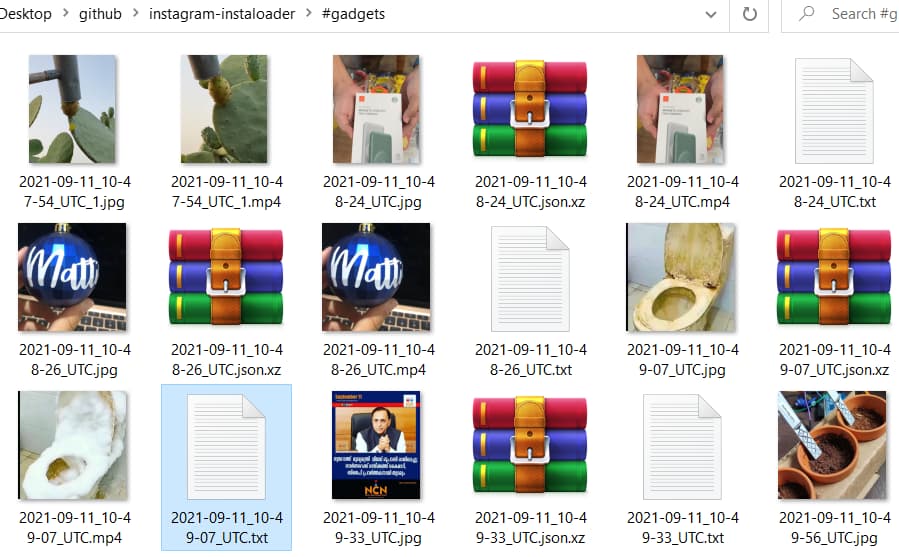
Python 有很多非常棒的开源工具软件包可以抓取互联网上的页面,甚至包括网页中的图片以及视频。
今天来给大家介绍一款名为 instaloader 的 Python 开源工具,使用它能够从 Instagram 中提取并下载视频、图像、个人资料头像、帖子和评论等数据,并允许自定义筛选你感兴趣的媒体和元数据。非常强大!
instaloader 主要功能:
- 下载个人资料、标签、用户故事、摘要和保存的媒体。
- 下载每个帖子下方的评论和地理位置标签。
- 自动检测配置文件名称的变更,并重命名目标目录。
- 允许对过滤器进行细粒度定制,以及自定义下载资料的存储位置。
- 遍历抓取及下载个人账户中所有图片和视频,以及个人头像(需登陆对应 instagram 账户)。
安装 instaloader
请在终端执行命令:
pip3 install instaloader
遍历抓取 instagram 账户内所有内容
安装完成后,请创建一个名为 instasave.py
的 Python 文件 ,然后输入以下代码:
import instaloader
from datetime import datetime
from itertools import dropwhile, takewhile
import csv
class GetInstagramProfile():
def __init__(self) -> None:
self.L = instaloader.Instaloader()
def download_users_profile_picture(self,username):
self.L.download_profile(username, profile_pic_only=True)
def download_users_posts_with_periods(self,username):
posts = instaloader.Profile.from_username(self.L.context, username).get_posts()
SINCE = datetime(2021, 8, 28)
UNTIL = datetime(2021, 9, 30)
for post in takewhile(lambda p: p.date > SINCE, dropwhile(lambda p: p.date > UNTIL, posts)):
self.L.download_post(post, username)
def download_hastag_posts(self, hashtag):
for post in instaloader.Hashtag.from_name(self.L.context, hashtag).get_posts():
self.L.download_post(post, target='#'+hashtag)
def get_users_followers(self,user_name):
'''Note: login required to get a profile's followers.'''
self.L.login(input("input your username: "), input("input your password: ") )
profile = instaloader.Profile.from_username(self.L.context, user_name)
file = open("follower_names.txt","a+")
for followee in profile.get_followers():
username = followee.username
file.write(username + "\n")
print(username)
def get_users_followings(self,user_name):
'''Note: login required to get a profile's followings.'''
self.L.login(input("input your username: "), input("input your password: ") )
profile = instaloader.Profile.from_username(self.L.context, user_name)
file = open("following_names.txt","a+")
for followee in profile.get_followees():
username = followee.username
file.write(username + "\n")
print(username)
def get_post_comments(self,username):
posts = instaloader.Profile.from_username(self.L.context, username).get_posts()
for post in posts:
for comment in post.get_comments():
print("comment.id : "+str(comment.id))
print("comment.owner.username : "+comment.owner.username)
print("comment.text : "+comment.text)
print("comment.created_at_utc : "+str(comment.created_at_utc))
print("************************************************")
def get_post_info_csv(self,username):
with open(username+'.csv', 'w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
posts = instaloader.Profile.from_username(self.L.context, username).get_posts()
for post in posts:
print("post date: "+str(post.date))
print("post profile: "+post.profile)
print("post caption: "+post.caption)
print("post location: "+str(post.location))
posturl = "https://www.instagram.com/p/"+post.shortcode
print("post url: "+posturl)
writer.writerow(["post",post.mediaid, post.profile, post.caption, post.date, post.location, posturl, post.typename, post.mediacount, post.caption_hashtags, post.caption_mentions, post.tagged_users, post.likes, post.comments, post.title, post.url ])
for comment in post.get_comments():
writer.writerow(["comment",comment.id, comment.owner.username,comment.text,comment.created_at_utc])
print("comment username: "+comment.owner.username)
print("comment text: "+comment.text)
print("comment date : "+str(comment.created_at_utc))
print("\n\n")
if __name__=="__main__":
cls = GetInstagramProfile()
#cls.download_users_profile_picture("best_gadgets_2030")
#cls.download_users_posts_with_periods("best_gadgets_2030")
#cls.download_hastag_posts("gadgets")
#cls.get_users_followers("best_gadgets_2030")
#cls.get_users_followings("best_gadgets_2030")
#cls.get_post_comments("laydline")
cls.get_post_info_csv("coolest.gadget")
由于以上代码涉及遍历抓取用户所有数据,所以您需要输入对应用户账户用户名和密码才可进行操作(您可以用作自己账户的数据备份)。
以上代码主要功能:
- 获取用户个人照片
- 遍历下载用户的帖子数据,如图片、视频等
- 下载标签帖子数据
- 获取该用户的所有粉丝名单
- 获取该用户所有关注者名单
- 获取用户帖子的评论
- 获取用户的所有帖子数据及相关评论
当您调用 download_hastag_posts(“gadgets”)
函数时,上述代码会自动创建标签 #gadgets
对应的文件夹,并将该标签所有相关内容保存在目录中。
如下图所示:
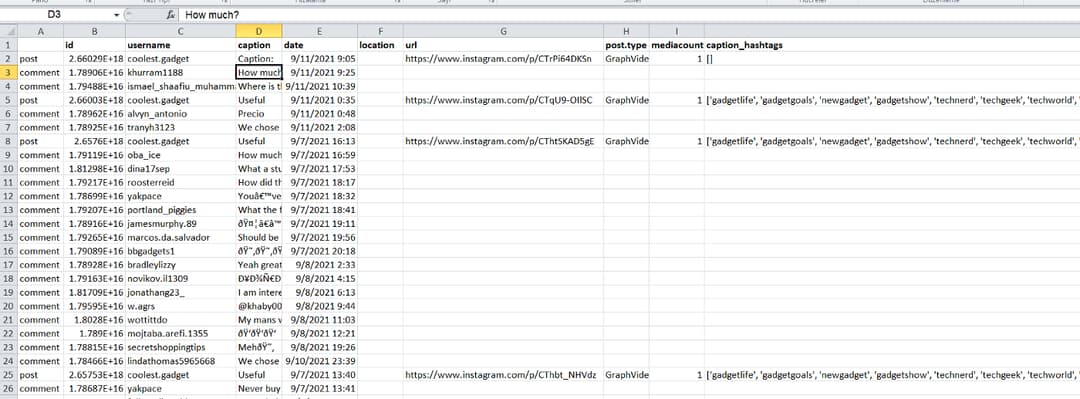
抓取并下载单个 instagram 视频(包括 IGTV 及 Reels )
您可以指定某个帖子链接,单独抓取其中的 IGTV 及 Reels 视频。本项操作无需登陆账号。
获取对应帖子ID
instagram 帖子 URL 样式为:
- 图片 或者 Reels:/p/CggLYswBrrx/ ,其对应帖子 ID 即为
CggLYswBrrx
- IGTV: /tv/Bq0OCtth_aQ/ ,其对应帖子 ID 即为
Bq0OCtth_aQ
下载对应视频
请在终端执行以下命令:
instaloader -- -帖子 ID
例如下载上述例子中 Reels 视频,请执行命令:
instaloader -- -CggLYswBrrx
更多命令参数请见:Command Line Options — Instaloader documentation
instaloader 项目地址
GitHub - instaloader/instaloader: Download pictures (or videos) along with their captions and other metadata from Instagram.
Download pictures (or videos) along with their captions and other metadata from Instagram. - instaloader/instaloader