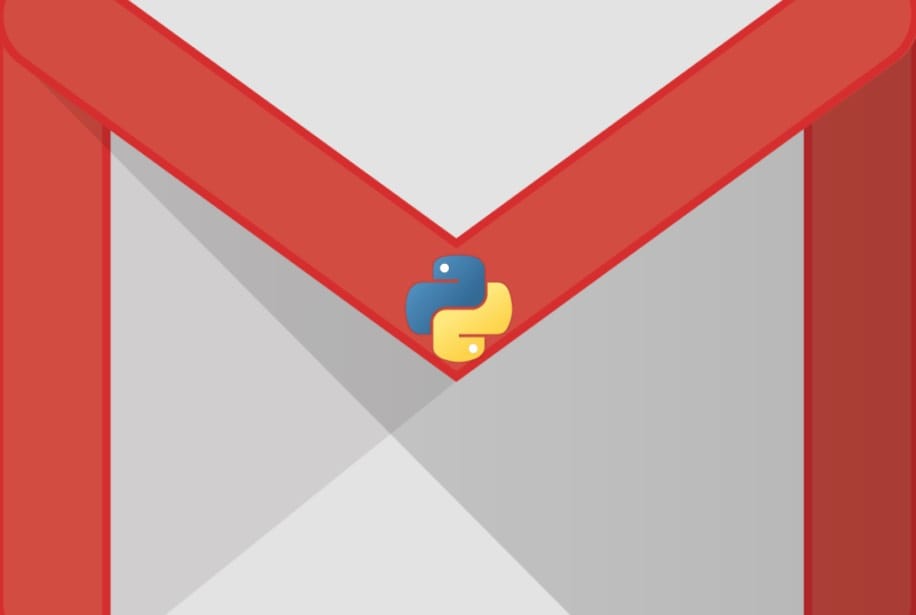
生活中我们经常遇到这样的情况,在我们注册一个网站或者服务的时候,需要使用一个电子邮箱来接收验证信息。然而,您可能只是临时需要使用该服务一次,今后不打算继续使用。或者,您不想将个人常用邮箱太多暴露于网络。在这种情况下,临时邮箱是最有效的解决方案。这些临时的一次性电子邮件地址可以用来替换您的常用电子邮件地址,并在超过一定期限后自动过期。
虽然有许多在线服务可以帮您创建临时电子邮件地址,但是,在本教程中,我将教您如何使用 Python 创建自己的临时 Gmail 邮箱地址并使用该地址接收电子邮件。
引入 Python 库
在这里我们将仅使用 2 个 Python 库,即 re
和 request
。如果您尚未安装它们,请使用以下命令安装它们。
pip3 install request
pip3 install re
然后引入这两个库:
import request
import re
生成 Gmail 邮箱地址
首先,我们需要使用一个免费 API 接口 Rapid API:
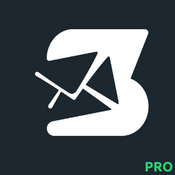
您需要注册该服务并免费获得一个 API KEY,我们需要使用该私钥自动生成Gmail邮箱账号。
然后,您可以使用以下代码生成一个新的 Gmail 邮箱地址并打印出该邮箱对应的账户密码。(请使用上面申请到的 API KEY 替换下方代码的 YOUR PRIVATE KEY
区域)
def generate_gmail(ID: int):
# access the API
url = "https://temp-gmail.p.rapidapi.com/get"
querystring = {"id":ID,"type":"alias"}
headers = {
'x-rapidapi-host': "temp-gmail.p.rapidapi.com",
'x-rapidapi-key': "YOUR PRIVATE KEY"
}
# send a request to the API
response = requests.request("GET", url, headers=headers, params=querystring)
# convert the response to JSON format
json_response = response.json()
# get gmail address
gmail = json_response['items']['username']
# get gmail password
password = json_response['items']['key']
print('Gmail address: %s' % str(gmail))
print('Password: %s' % str(password))
输入示例:
generate_gmail(ID=77)
输出示例:
Gmail address: [email protected]
Password: ***********T091ngKLOOPdXhfVCiHHDWlE16Ds
请注意,输入的“ID”必须是一个整数,对应于 API 数据集中的特定 Gmail 地址(包含 1000 个 Gmail)。如果您更改“ID”的值,该函数将生成不同的 Gmail 地址。
检查收件箱
好的,现在我们已经创建了一个临时的 Gmail 地址,接下来,我们来检查其收件箱——可以使用check_inbox(gmail: str, password: str)
函数来检查邮箱中新邮件。
该函数会将会打印出新邮件 message_id
、发件人姓名、日期、时间和主题,如果邮箱中没有新邮件,则返回收件箱为空提示。
代码如下:
def check_inbox(gmail: str, password: str):
# access the API
url = "https://temp-gmail.p.rapidapi.com/check"
querystring = {"username":gmail,"key":password}
headers = {
'x-rapidapi-host': "temp-gmail.p.rapidapi.com",
'x-rapidapi-key': "YOUR PRIVATE KEY"
}
# send a request to the API
response = requests.request("GET", url, headers=headers, params=querystring)
# convert the response to JSON format
json_response = response.json()
# print the message from the API
print('API message: %s' % str(json_response['msg']))
# check whether the inbox is empty or not
if json_response['items'] == []:
print("inbox is empty")
# if the inbox is not empty, print the details of the newest mail
else:
message_id = json_response['items'][0]['mid']
print('Message ID: %s' % str(message_id))
print('From: %s' % str(json_response['items'][0]['textFrom']))
print('Date: %s' % str(json_response['items'][0]['textDate']))
print('Subject: %s' % str(json_response['items'][0]['textSubject']))
输入示例:
MyGmail = "[email protected]"
MyPassword = "***********T091ngKLOOPdXhfVCiHHDWlE16Ds"
check_inbox(gmail=MyGmail, password=MyPassword)
输出示例:
Message ID: 21e5dd2934sdfd11
From: Amir Ali Hashemi
Date: 2022–01–15 20:03:22
Subject: TEST EMAIL
接下来,我们将需要使用 message_id
来获取邮件正文。
读取邮件正文
为了读取邮件正文,我们需要使用 Gmail 地址以及该邮件对应的 message_id
,向 API 发送请求。API 会以 HTML 格式返回正文内容,并附带 HTML 标记。
邮件正文示例:
<div dir=”ltr”><div>这是一条来自啁啾集的测试邮件</div><div><br></div></div>
为便于阅读,我们使用该 re
库来删除 HTML 标签并打印出清理后的纯文本正文内容。
代码如下:
def read_message(gmail: str, message_id: str):
# access the API
url = "https://temp-gmail.p.rapidapi.com/read"
querystring = {"username":gmail,"message_id":message_id}
headers = {
'x-rapidapi-host': "temp-gmail.p.rapidapi.com",
'x-rapidapi-key': " YOUR PRIVATE KEY"
}
# send a request to the API
response = requests.request("GET", url, headers=headers, params=querystring)
# convert the response to JSON format
json_response = response.json()
# remove the html tags from the body of the mail
body_message = re.sub(r'<.*?>', '', json_response['items']['body'])
print('Body message: %s' % str(body_message))
输入示例:
MyGmail = '[email protected]'
MessageID = '21e5dd2934sdfd11'
read_message(gmail=MyGmail, message_id=MessageID)
输出示例:
Body message: 这是一条来自啁啾集的测试邮件
恢复 Gmail 邮箱
如本文开头所述,临时电子邮件会在一定时间期限后过期(通常在 10 分钟后),这就意味着密码将被重置!
因此,如果您创建了一个 Gmail 临时邮箱并希望在一段时间后重新访问它,则需要对其进行恢复。
您可以使用 restore_gmail(gmail: str)
函数来通过恢复您之前生成的 Gmail 邮箱来获取新密码,以便可以重复使用该邮箱。
代码如下:
def restore_gmail(gmail: str):
# access the API
url = "https://temp-gmail.p.rapidapi.com/restore"
querystring = {"username":gmail}
headers = {
'x-rapidapi-host': "temp-gmail.p.rapidapi.com",
'x-rapidapi-key': " YOUR PRIVATE KEY"
}
# send a request to the API
response = requests.request("GET", url, headers=headers, params=querystring)
# convert the response to JSON format
json_response = response.json()
print('Gmail address: %s' % str(json_response['items']['username']))
print('Password: %s' % str(json_response['items']['key']))
输入示例:
restore_gmail(‘[email protected]’)
输出示例:
Gmail address: [email protected]
Password: *******************m5nLnoGbR094ngJCCITedTTsddiEE65Es
总而言之,临时邮件不仅可以给个人的日常使用带来极大的便利,还可以集成到项目中完成更多复杂功能,以后我再展开来谈谈这部分。